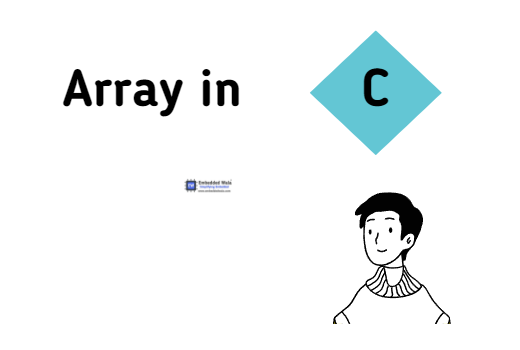
Arrays are a fundamental data structure in programming that allows to store a collection of variables of the same data type. In C, arrays are used extensively for data manipulation and processing. In this blog, basics of arrays in C, how to declare and initialize them, and how to access and manipulate array elements are captured.
Declaring and Initializing Arrays
In C, an array can be declared by specifying its data type and size, as shown in the following example:
int myArray[5];
This creates an integer array named myArray
with a size of 5. By default, all elements in the array are initialized to 0.
An array can also be initialized when it is declared by assigning a list of values to the elements, as shown in the following example:
int myArray[] = {1, 2, 3, 4, 5};
This creates an integer array named myArray
with a size of 5 and initializes its elements to the values 1, 2, 3, 4, and 5.
Accessing Array Elements
User can access individual elements in an array by their index, which starts from 0. For example, to access the first element in myArray
, following code can also be used:
int firstElement = myArray[0];
Similarly, to access the third element, the following code would be used:
int thirdElement = myArray[2];
Modifying Array Elements
In an array, individual element can be modified by assigning a new value to the element’s index, as shown in the following example:
myArray[0] = 10;
This changes the value of the first element in myArray
from 1 to 10.
Iterating Over Arrays
User can iterate over the elements in an array using a loop. For example, the following code prints all the elements in myArray
:
for (int i = 0; i < 5; i++) { printf("%d\n", myArray[i]); }
This loops through each element in myArray
and prints its value to the console.
Multi-Dimensional Arrays In C
Multi-dimensional arrays can be created by declaring an array of arrays. For example, the following code declares a 2-dimensional integer array with a size of 3×3:
int myArray[3][3] = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
This creates a 2-dimensional integer array named myArray
with 3 rows and 3 columns.
To access an individual element in a multi-dimensional array, two indices can also be used, as shown in the following example:
int element = myArray[1][2];
This accesses the element in the second row and third column of myArray
. Below share code is showcasing the usage of an array in C.
#include <stdio.h> int main() { // declare and initialize an integer array with a size of 5 int myArray[5] = {1, 2, 3, 4, 5}; // access the first element in myArray int firstElement = myArray[0]; printf("First element: %d\n", firstElement); // modify the third element in myArray myArray[2] = 10; printf("Modified array: "); for (int i = 0; i < 5; i++) { printf("%d ", myArray[i]); } printf("\n"); // create a 2-dimensional integer array with a size of 3x3 int my2DArray[3][3] = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }; // access the element in the second row and third column of my2DArray int element = my2DArray[1][2]; printf("Element at (1, 2): %d\n", element); return 0; }
This code first declares and initializes an integer array with a size of 5, and then accesses the first element and modifies the third element. It then creates a 2-dimensional integer array with a size of 3×3, and accesses an element in the second row and third column of the array.
When the code is executed, the output will be:
First element: 1 Modified array: 1 2 10 4 5 Element at (1, 2): 6
This output shows that the first element in the myArray
is accessed correctly and the third element is modified from 3 to 10. The output also shows that the element at (1, 2) in my2DArray
is accessed correctly and is equal to 6.
At the end, it can be concluded that Arrays are a fundamental data structure in programming that allow you to store multiple values of the same data type in a single variable. In C, arrays are used extensively for data manipulation and processing. In this blog, we explored the basics of arrays in C, including how to declare and initialize them, how to access and modify array elements, how to iterate over arrays, and how to create multi-dimensional arrays.